Checking if a String starts with specific characters in Client Scripts in ServiceNow
Two alternative methods to JavaScript String startWith() for ServiceNow Client Scripts.
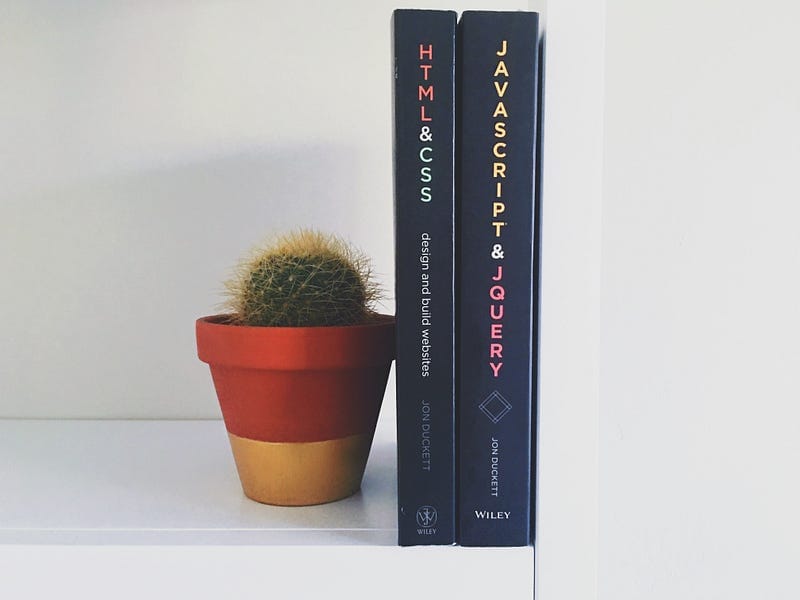
Sometimes when we work in ServiceNow Client Scripts, we have to check a field in a form with a set of characters they start with. If we work in web development, we could use the startWith() method introduced in JavaScript ES6 (ECMAScript 2015). A simple and easy-to-use method allows for checking the prefix of a String and returns a Boolean value true or false based on whether the given string begins with the specified letter or word. At the time of writing this post, ServiceNow does not support the use of this method, so generally, when I have to implement a requirement where I need a function like startWith(), I use these two alternatives, which allows me to accomplish the same output, the first one isindexOf()
and the second is substring().
Before, we talk about the indexOf() and substring(), let’s first understand how we use startWith() in modern JavaScript.
startWith() syntax:
str.startsWith(searchString[, position])
In this example, we have declared a variable called str
using const,
the keyword const is also ECMAScript 2015. We assign the string ‘JavaScript is great’. We can test it using the browser console or my favourite JS snippet tester JSBin.const str = ‘JavaScript is great’;
Then, we use console.log to log to the browser debugging console and startWith()
to determine if the string begins with the specified string. This method returns true
if the string starts with the specified characters and, false
if not, keeping in mind that this is case-sensitive.
In this first, the expected output is true since the specified string matches the value stored in str.
console.log(str.startsWith(‘JavaScript is’)); //true
If the string does not begin with the specified characters, it returns false.console.log(str.startsWith(‘Java is’)); //false
Other examples for false return on line 4 and 5:const str = ‘JavaScript is great’;
console.log(str.startsWith(‘JavaScript is’)); //true
console.log(str.startsWith(‘Java is’)); //false
console.log(str.startsWith(‘Python is’, 3)); //false
Now, how to do the above in ServiceNow using substring(). We want to add a field message to the Short Description field if the Short Description starts with the word “SAP.”
substring() syntax:string.substring(start_position [, end_position]);
The start_position is the position in the string where the extraction will start. The first position in the string is 0 and end_position 3, for the word “SAP” separating as follows /S/A/P, being zero before the “S” If this parameter is not provided, the substring() method will return all characters to the end of the string.
First, let’s declare a variable to assign the value of a short description. This is on an onChange type of Client Script based on Short Description.var shortDesc = g_form.getValue('short_description');
Then, we use conditional if
and substring()
, we specify the start and end of the string, for the word SAP, in this case (0, 3).if (shortDesc.substring(0, 3) == 'SAP') {
//do something if true
}
Showing the field message if true.g_form.showFieldMsg('short_description', 'SAP Incident Ticket');
The complete Client Script:g_form.showFieldMsg('short_description', 'SAP Incident Ticket');function onChange(control, oldValue, newValue, isLoading, isTemplate) {
if (isLoading || newValue === '') {
return;
}
var shortDesc = g_form.getValue('short_description');if (shortDesc.substring(0, 3) == 'SAP') {
g_form.showFieldMsg('short_description', 'SAP Incident Ticket');
}
}
Now, let’s work with indexOf(). When we work manipulating a string, it is quite frequent that we need to know if a particular element exists and in which position is. Of course, we can create a method that goes through all the contained elements, but indexOf() was purposely built to save us time.
In browser console or JSbin, we try the following script.var str = "JavaScript is great!";
console.log(str.indexOf("JavaScript")); // return 0
console.log(str.indexOf("Python"));// return -1
In the above example, if the short description matches, it will return 0, if not, -1, we can use this output for our client script as well. First, we declare our variable shortDesc
and assign the value of the Short Description field.var shortDesc = g_form.getValue(‘short_description’);
Then, we use an if statement to check whether the condition is true, making use of indexOf().
if ((shortDesc.indexOf(‘SAP’)) == 0) { //return true
//do something
}
For this particular demo, we are showing a message below the short description field.g_form.showFieldMsg(‘short_description’, ‘SAP Incident Ticket’);
The complete Client Script using indexOf () to check if short description starts with “SAP”.function onChange(control, oldValue, newValue, isLoading, isTemplate) {
if (isLoading || newValue === '') {
return;
}
var shortDesc = g_form.getValue('short_description');
if ((shortDesc.indexOf('SAP')) == 0) {
g_form.showFieldMsg('short_description', 'SAP Incident Ticket');
}
}
Final Notes:
There are many built-in methods in JavaScript that help us work with Strings. These two substring () and indexOf() can be used as an alternative to startWith(). Recapping, the substring() method gets a part of the original string and returns it as a new string, indexOf() method works; differently, this method returns the position of the first occurrence of a specified value in a string and returns -1 if the value to search for never occurs.