Creating an Array with Affected CIs in ServiceNow
Adding a list of CI names into an array using the JavaScript push() method.
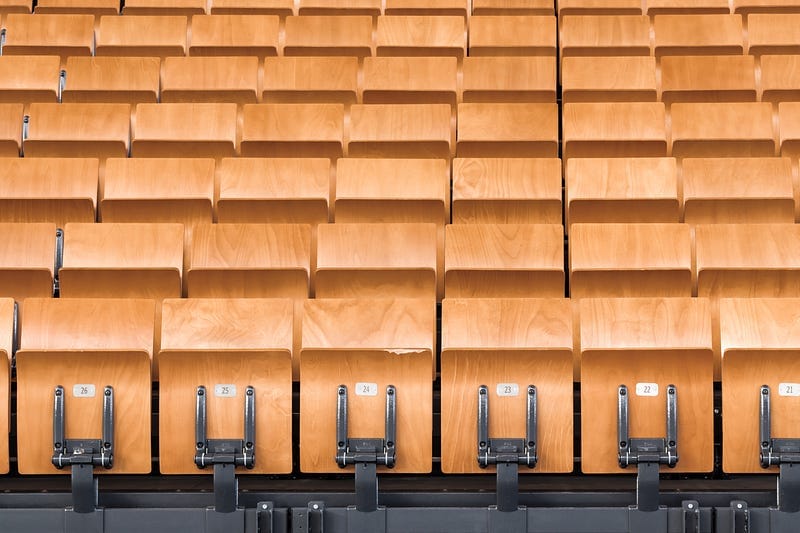
Knowing how to create an array of elements from a table in ServiceNow can come in handy when working on integrations with third-party applications.
Let’s suppose that we have an incident record and the affected CIs for the incident. We want to populate a string type field u_affected_cis
with the list of affected CIs for the incident.
To implement this, we create an after Business Rule in the task_ci table that runs on create and delete. So, for every new or deletion of affected CIs, the system will update the incident field u_affected CIs with the refreshed list of CI names.
First, we declare ciList using Array literal syntax.var ciList = [];
Then, we create a GlideRecord to query the task_ci table. GlideRecord Creates an instance of the GlideRecord class for the task_ci table.var gr = new GlideRecord('task_ci');
Then, we query for the table task_ci.gr.addQuery('task', current.task);
We iterate through the list of affected CIs from the result of the query. The toString() method returns the value of a String object, and the push() method adds new items to the end of an array.
var gr = new GlideRecord('task_ci');
gr.addQuery('task', current.task);
gr.query();
while (gr.next()) {
var affectedCIs = gr.ci_item.name.toString();
ciList.push(affectedCIs);
}
Once we have filled our ciList array with elements from CI names. We query the table incident where sys_id = current deleted or added task_ci record and populate the field u_affected_cisvar gr2 = new GlideRecord('incident');
gr2.addQuery('sys_id', current.task);
gr2.query();
if (gr2.next()) {
gr2.u_affected_cis = ciList.toString();
gr2.update();
}
The complete Business Rules.(function executeRule(current, previous /*null when async*/ ) {
var ciList = [];
var gr = new GlideRecord(‘task_ci’);
gr.addQuery(‘task’, current.task);
gr.query();
while (gr.next()) {
var affectedCIs = gr.ci_item.name.toString();
ciList.push(affectedCIs); //Add new items to the end of the array
}
var gr2 = new GlideRecord(‘incident’);
gr2.addQuery(‘sys_id’, current.task);
gr2.query();
if (gr2.next()) {
gr2.u_affected_cis = ciList.toString(); //populate u_affected_cis
gr2.update();
}
})(current, previous);
Key Notes
push()
method adds new items to an array and returns the new length. The method adds the new items at the end of the array. This method changes the length of the array.